Melissa at gilatrailsinfo.org does an amazing job at documenting and improving trails in the Gila. If you ever get a chance to join one of her trail projects, do so, you will learn a lot! She keeps a map of recent work on the trails, and shows trails that should be avoided, whether due to recent burns, neglect, or other issues. Trails to avoid are in red, and you can see several are flagged in a couple of areas.
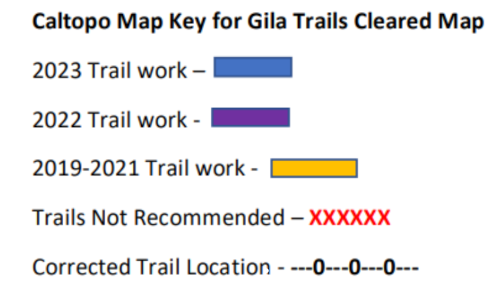
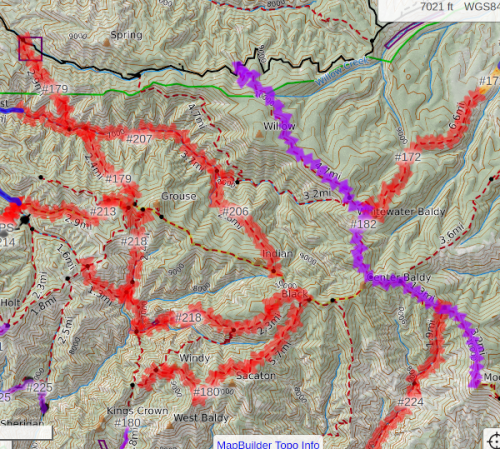
How do we modify our graph to avoid the not-recommended trails, without doing a huge amount of manual work? My approach will be to make a text list of banned trails, and then write a function that searches for those trail names in the OSMnx edge attributes of our graph, and then delete those edges. One potential drawback is that the entire trail with that name will be deleted, even if only a segment should be closed, but perhaps this will be close enough for a first approximation.
Another approach might be to change the weight of not-recommended trails, so that they might be used, but not often. For now, let us delete them altogether, to reduce the potential of erosion on damaged trails.
not_recommended = ['deloche and winn canyon', #179 'catwalk', #207 bummer 'redstone', #206 'spider creek', 'little dry', #180 'west fork mogollon', #224 'trail canyon', #169 '#158', #158 'ring canyon', #162 'iron creek', #172 'golden link', #218 '#153', #153 mogollon trail '#217', #217 '#709', #709 east fork private property ######## aldo side 'aspen mountain', #814 '#73', #73 # keep this one for now 'black range crest', #79 'middle fork mimbres', #78 'sids prong', #121 '#120', #120 ]
Here is a simple routine to remove these not-recommended trails from our graph:
edges_to_remove = [] trails_removed = set() for u,v,k,d in J.edges(keys=True,data=True): if 'name' in d: trail_name = d['name'] trail_name = trail_name.lower() if any(e for e in not_recommended if e in trail_name): edges_to_remove.append( (u,v,k) ) trails_removed.add(trail_name) J.remove_edges_from(edges_to_remove) print('trails removed: ', trails_removed)
Here is the total mileage change from deleting these trails.
#before
total length 1579.62 miles in largest subgraph
#after
total length 1451.61 miles not-recommended trails removed
Trails should be deleted before the graph is simplified, because simplification concatenates several road and trail names on certain routes.
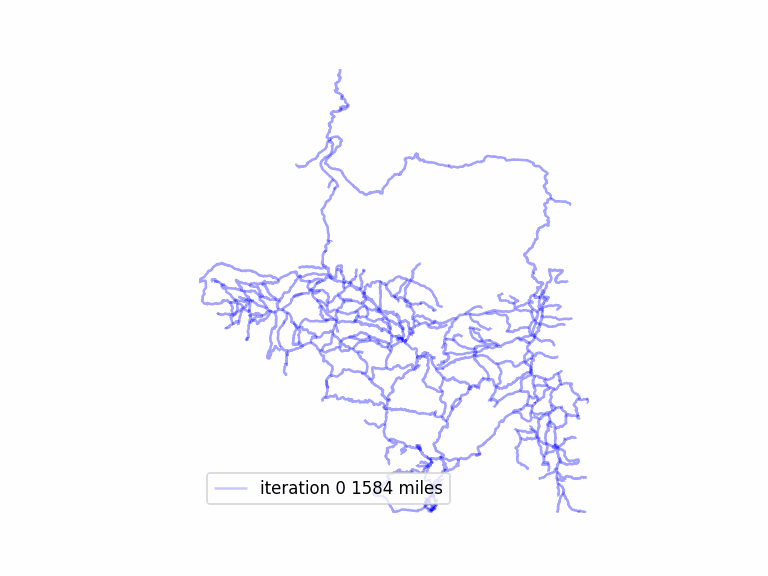
Download source code here.