Let us create a small imaginary trail graph in Python with NetworkX, and play around with plotting and paths. Suppose we have a graph with nodes from 0 to 15:
import networkx as nx import matplotlib.pyplot as plt G=nx.Graph() edges=[(0,1,1),(1,2,1),(2,3,1),(3,4,1),(4,5,1),(5,6,1),(6,7,1), (8,3,2),(9,4,1),(9,5,1),(10,7,2), (8,9,2),(9,10,2), (8,11,2),(12,10,2), (11,12,2),(12,13,2),(13,7,2), (11,14,2),(14,15,4),(15,13,1)] G.add_weighted_edges_from(edges)
The tuple (8,11,2) means an edge goes from node 8 to node 11, with weight 2.
Now we specify where the nodes are located.
#pos=nx.spring_layout(G) does not give satisfactory results. # Draw node positions the hard way. pos=[(0,4),(1,4),(2,4),(3,4),(4,4),(5,4),(6,4),(7,4), (1,3),(3,3),(5,3), (3,2),(6,2),(8,2), (4,1),(7,1)]
We are almost ready to draw the network. Since we want to draw weights for the edges, we have an extra step.
options = { "font_size": 16, "node_size": 1000, "node_color": "white", "edgecolors": "black", "linewidths": 5, "width": 5, } nx.draw_networkx(G, pos,**options) labels = {e: G.edges[e]['weight'] for e in G.edges} nx.draw_networkx_edge_labels(G, pos, edge_labels=labels, font_color="red") plt.show()
And here is our result.
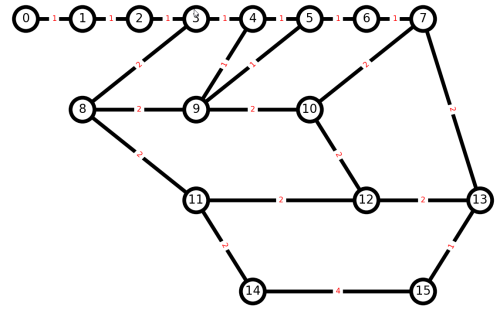
Think how we can convert this into a Euler graph, so all nodes have an even number of edges. We can try a Networkx library function, but should not expect it will do what we want, because it will create a multigraph, with more than one edge between nodes.
Continue reading “Trails and Graph Theory 3: NetworkX”